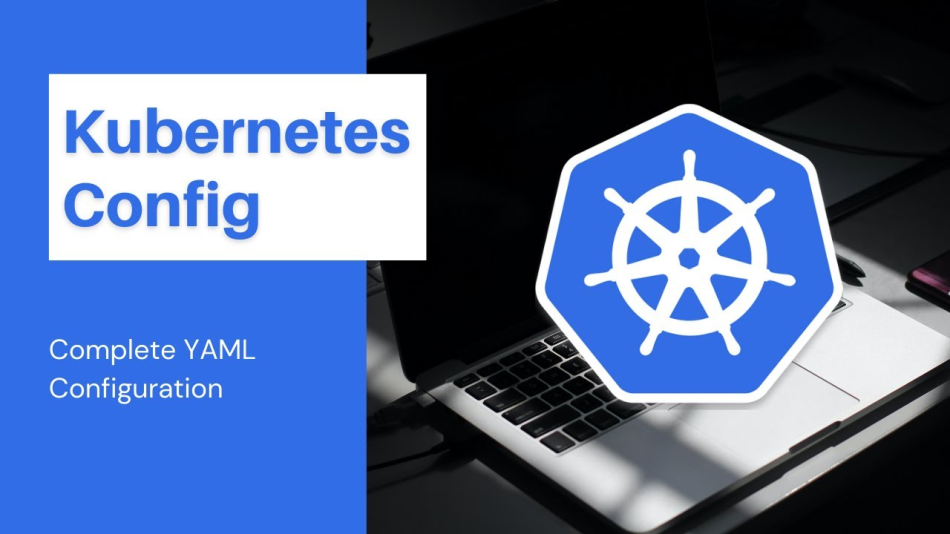
Managing Configuration in Kubernetes: Understanding ConfigMaps and Secrets
Kubernetes, a powerful orchestration platform for containerized applications, offers several essential tools for managing configurations. Among these, ConfigMaps and Secrets stand out for managing non-sensitive and sensitive information, respecti...
1. What are Kubernetes ConfigMaps and Secrets?
Before diving deep into examples and demos, let's understand what ConfigMaps and Secrets are in Kubernetes and how they play a crucial role in managing application configuration.
1.1 What is a ConfigMap?
A ConfigMap is a Kubernetes object that allows you to store non-sensitive key-value pairs, which can be accessed by your applications running inside the cluster. ConfigMaps are generally used to store configuration settings such as environment variables, command-line arguments, and configuration files.
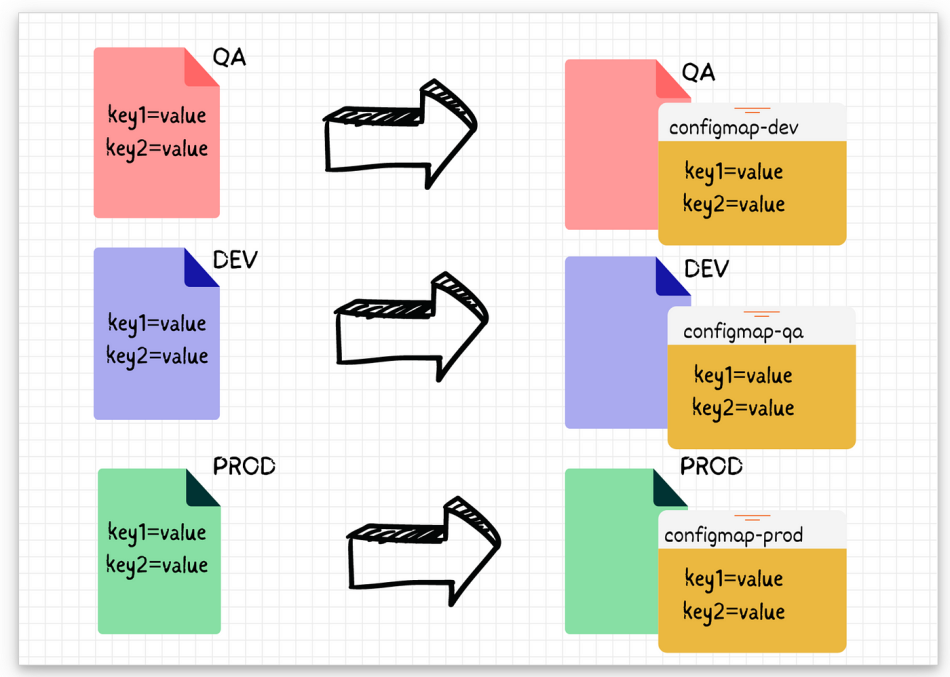
Example:
A simple example of a ConfigMap might look like this:
apiVersion: v1
kind: ConfigMap
metadata:
name: app-config
data:
APP_ENV: "production"
APP_DEBUG: "false"
Here, app-config is the ConfigMap that contains two key-value pairs: APP_ENV and APP_DEBUG.
1.2 What is a Secret?
Kubernetes Secrets are similar to ConfigMaps, but they are specifically designed for storing sensitive information such as passwords, API keys, and TLS certificates. Secrets are stored in base64 encoded format and are generally more secure than ConfigMaps.
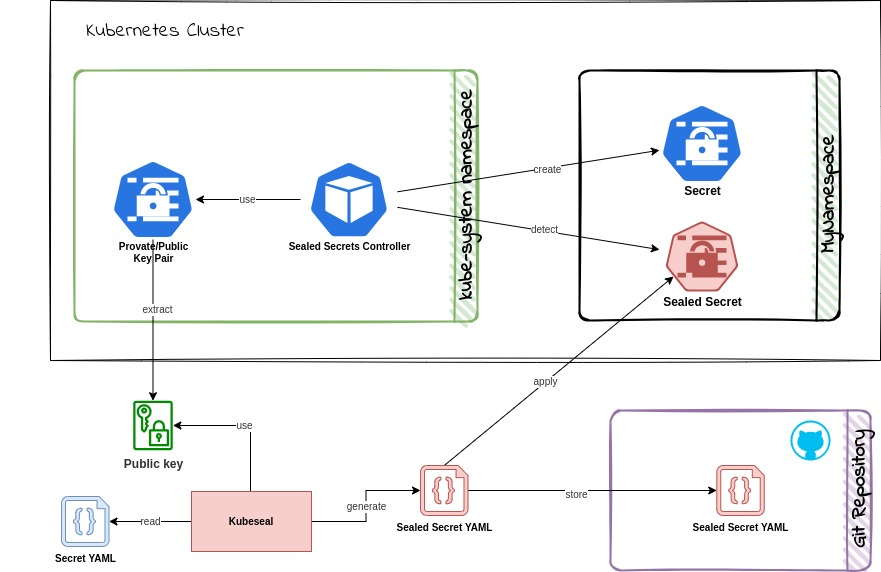
Example:
A basic example of a Secret might look like this:
apiVersion: v1
kind: Secret
metadata:
name: db-secret
type: Opaque
data:
username: YWRtaW4=
password: cGFzc3dvcmQ=
1.3 Differences Between ConfigMaps and Secrets
While both ConfigMaps and Secrets are used for storing configuration data, there are key differences between them:
- Purpose: ConfigMaps are used for non-sensitive data, while Secrets are for sensitive data.
- Storage: Secrets are stored in an encrypted format, whereas ConfigMaps are stored in plain text.
- Encoding: Secrets use base64 encoding for data storage, while ConfigMaps store data in plain text.
2. Behind the scene
2.1 ConfigMap
ConfigMaps allow you to decouple configuration artifacts from the container image, enabling you to dynamically update configuration without rebuilding the container images.
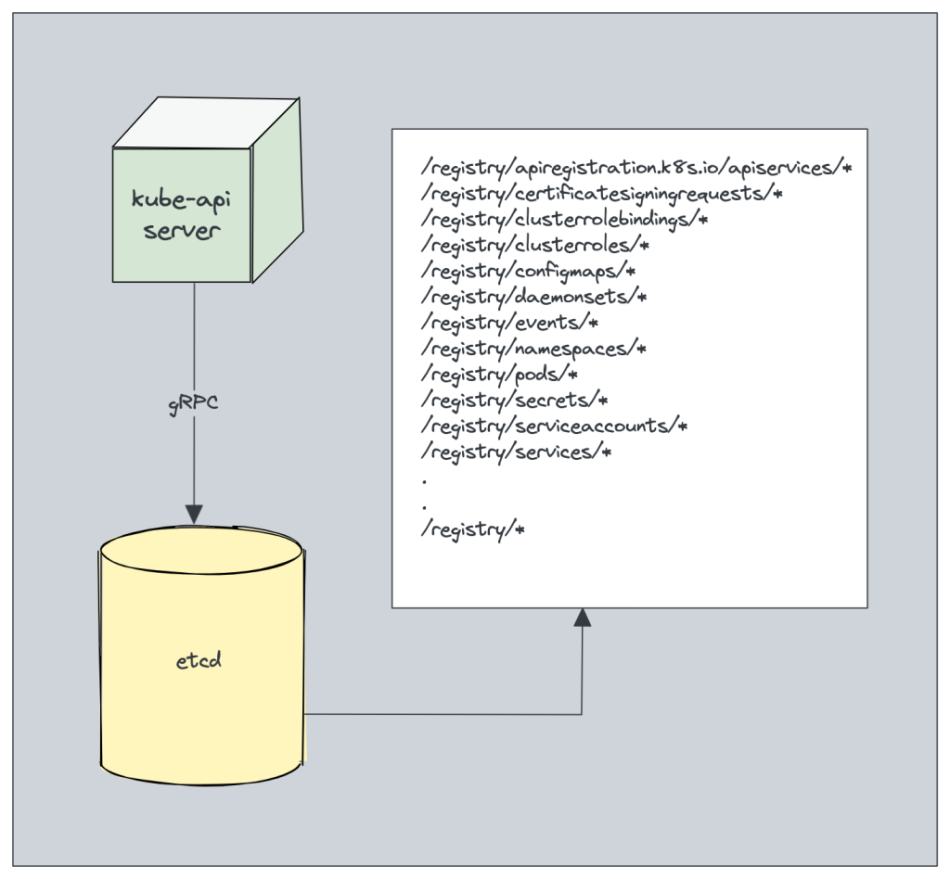
How Kubernetes Stores ConfigMaps
- Etcd Database: Kubernetes stores ConfigMaps, like most of its cluster data, in etcd, a distributed key-value store that is the primary backing store for all Kubernetes cluster data. Etcd is a highly consistent and reliable storage that Kubernetes uses to manage the state of the cluster.
- Namespace and Object Metadata: Each ConfigMap is stored within a specific namespace in the Kubernetes cluster. It also has associated metadata such as name, namespace, labels, and annotations to help identify and manage the ConfigMap.
- Data Serialization: When a ConfigMap is created, it is serialized into a JSON or YAML format and stored as part of the Kubernetes API objects. This serialized data is stored in etcd in a key-value format, where the key represents the unique path to the resource (e.g., /registry/configmaps/{namespace}/{name}) and the value contains the serialized JSON or YAML representation of the ConfigMap.
- Access Control and Security: Access to ConfigMaps is controlled via Kubernetes Role-Based Access Control (RBAC). This ensures that only authorized users and services can create, read, update, or delete ConfigMaps within a particular namespace.
Where ConfigMaps Are Stored
- Location in etcd: The ConfigMaps are stored in etcd, the primary datastore for Kubernetes. They are typically stored under a path structure like /registry/configmaps/{namespace}/{configmap-name}.
- Cluster State Management: Etcd serves as the source of truth for the state of the cluster, and ConfigMaps are stored along with other Kubernetes objects like Pods, Deployments, and Services. This centralized storage approach helps ensure that the cluster state is consistent and recoverable.
2.2 Secret
Kubernetes Secrets provide a way to securely store and manage this sensitive data, reducing the risk of accidental exposure.
Kubernetes Secrets are stored in base64-encoded format. While this is not encryption (base64 is a simple encoding), it makes the data less human-readable. To ensure the security of secrets, additional encryption measures should be applied, such as encrypting secrets at rest in etcd.
Different Types of Secrets: Kubernetes supports different types of Secrets to cater to various use cases:
- Opaque: The default Secret type, used for storing arbitrary key-value pairs.
- kubernetes.io/dockerconfigjson: Used for storing Docker registry credentials, allowing pods to pull private images.
- kubernetes.io/tls: Used for storing TLS certificates and keys, typically for HTTPS connections.
- Service Account Token: Automatically created secrets that store tokens for service accounts.
Secrets are namespace-scoped, meaning they are only accessible within the namespace where they are created. Access to secrets is controlled by Role-Based Access Control (RBAC) policies, ensuring that only authorized users and services can access or modify secrets.
By default, Kubernetes stores secrets unencrypted in etcd. However, for enhanced security, Kubernetes supports encryption at rest for secrets. This ensures that even if etcd data is accessed, the secrets remain protected.
Secrets can be consumed by pods in several ways:
- As Environment Variables: Secrets can be exposed as environment variables to containers.
- As Volumes: Secrets can be mounted as files in a container’s filesystem, allowing applications to read them securely.
- As Image Pull Secrets: Used for pulling images from private container registries.
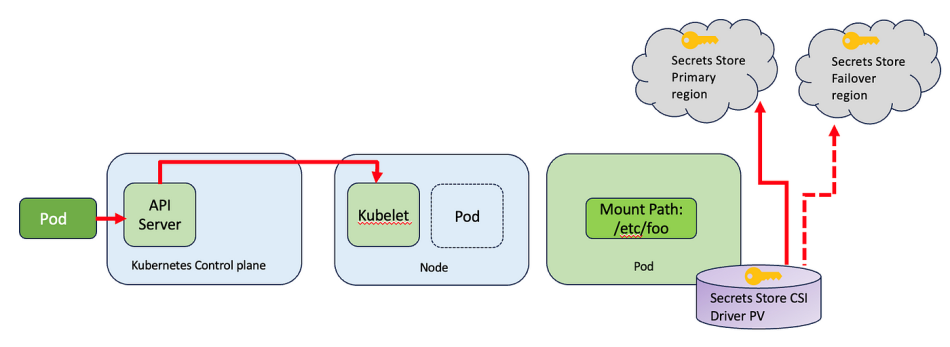
How Secrets Are Stored
- Etcd Storage: Just like ConfigMaps, Secrets are stored in the etcd database, which is the backend store for all Kubernetes cluster data. However, secrets should be encrypted at rest in etcd to protect sensitive information.
- Serialized in Base64: Secrets are serialized and base64-encoded when stored. The base64 encoding is primarily for data transport purposes and does not provide security.
3. How to Create and Use ConfigMaps in Kubernetes
Creating and using ConfigMaps in Kubernetes is straightforward. Below, we’ll explore the steps needed to create a ConfigMap and inject it into a Kubernetes Pod.
3.1 Creating a ConfigMap
There are several ways to create a ConfigMap in Kubernetes. The most common methods are using a YAML file or the kubectl command-line tool.
Method 1: Using a YAML File
The following YAML file defines a ConfigMap named app-config:
apiVersion: v1
kind: ConfigMap
metadata:
name: app-config
data:
APP_ENV: "production"
APP_DEBUG: "false"
To create the ConfigMap, apply the YAML file using the following command:
kubectl apply -f app-config.yaml
Method 2: Using kubectl Command
You can also create a ConfigMap directly from the command line:
kubectl create configmap app-config --from-literal=APP_ENV=production --from-literal=APP_DEBUG=false
3.2 Using ConfigMaps in Pods
Once the ConfigMap is created, it can be injected into a Pod as environment variables or mounted as a volume. Here’s an example of how to inject the app-config ConfigMap into a Pod:
apiVersion: v1
kind: Pod
metadata:
name: configmap-demo
spec:
containers:
- name: app-container
image: nginx
envFrom:
- configMapRef:
name: app-config
This Pod uses the app-config ConfigMap to set environment variables for the container.
3.3 Mounting ConfigMaps as Volumes
ConfigMaps can also be mounted as volumes to make configuration files available to applications inside the container. Here is an example:
apiVersion: v1
kind: Pod
metadata:
name: configmap-volume-demo
spec:
containers:
- name: app-container
image: nginx
volumeMounts:
- name: config-volume
mountPath: "/etc/config"
volumes:
- name: config-volume
configMap:
name: app-config
This Pod mounts the app-config ConfigMap as a volume at /etc/config.
4. How to Create and Use Secrets in Kubernetes
Creating and using Secrets in Kubernetes involves a few additional steps to ensure security.
4.1 Creating a Secret
Secrets can be created using YAML files or the kubectl command-line tool.
Method 1: Using a YAML File
Here’s an example of creating a Secret using a YAML file:
apiVersion: v1
kind: Secret
metadata:
name: db-secret
type: Opaque
data:
username: YWRtaW4=
password: cGFzc3dvcmQ=
To create the Secret, run:
kubectl apply -f db-secret.yaml
Method 2: Using kubectl Command
Alternatively, you can create a Secret from the command line:
kubectl create secret generic db-secret --from-literal=username=admin --from-literal=password=password
4.2 Using Secrets in Pods
Secrets can be injected into a Pod as environment variables or mounted as volumes, similar to ConfigMaps. Here’s an example of using db-secret in a Pod:
apiVersion: v1
kind: Pod
metadata:
name: secret-demo
spec:
containers:
- name: app-container
image: nginx
env:
- name: DB_USERNAME
valueFrom:
secretKeyRef:
name: db-secret
key: username
- name: DB_PASSWORD
valueFrom:
secretKeyRef:
name: db-secret
key: password
4.3 Mounting Secrets as Volumes
Secrets can also be mounted as volumes to access sensitive information directly as files. Here's how to do it:
apiVersion: v1
kind: Pod
metadata:
name: secret-volume-demo
spec:
containers:
- name: app-container
image: nginx
volumeMounts:
- name: secret-volume
mountPath: "/etc/secret"
volumes:
- name: secret-volume
secret:
secretName: db-secret
This Pod mounts the db-secret Secret as a volume at /etc/secret.
5. Best Practices for Using ConfigMaps and Secrets
Using ConfigMaps and Secrets effectively involves following best practices to ensure security and manageability.
Keep Secrets Encrypted
Although Kubernetes stores Secrets in base64 encoding by default, it is highly recommended to enable encryption at rest to enhance security.
Limit Access to Secrets
Control access to Secrets through Role-Based Access Control (RBAC) policies. Only allow necessary components or services to access specific Secrets.
Use Environment Variables Wisely
While environment variables are a convenient way to inject configuration data, avoid using them for highly sensitive data such as API keys or passwords. Instead, prefer mounting Secrets as volumes.
6. Conclusion
Understanding Kubernetes ConfigMaps and Secrets is fundamental for securely managing application configurations. ConfigMaps allow you to handle non-sensitive data, while Secrets provide a safe way to store sensitive information. By following the examples and best practices provided in this guide, you can manage configurations more securely and effectively in your Kubernetes environment.
Have questions or need further clarification? Feel free to leave a comment below!
Read more at : Managing Configuration in Kubernetes: Understanding ConfigMaps and Secrets