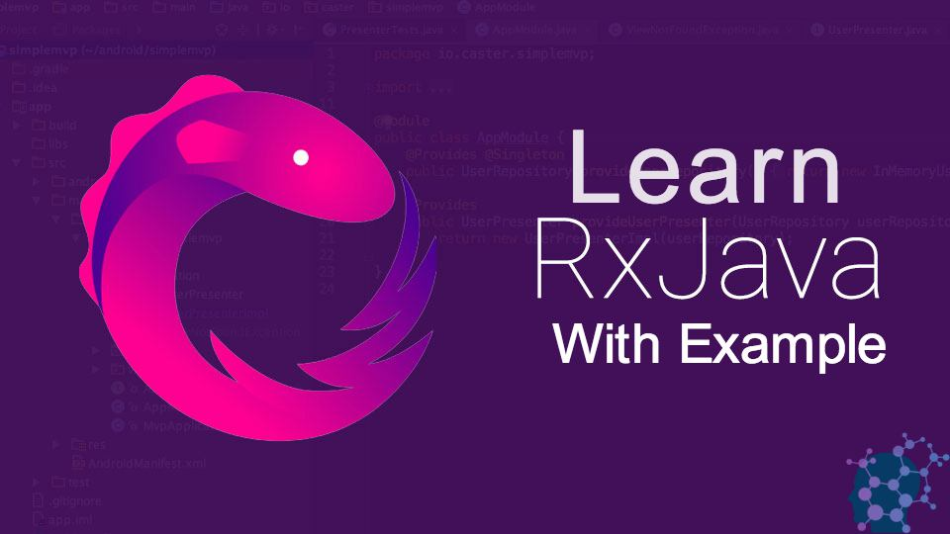
Secrets of RxJava: Why It’s the Key to Managing Asynchronous Operations in Java
Have you ever been bogged down by the complexity of managing asynchronous operations in Java? Perhaps you’ve found yourself writing endless callbacks, leading to unmaintainable and cluttered code. Or maybe you’re looking for a more elegant way to...
1. What is RxJava?
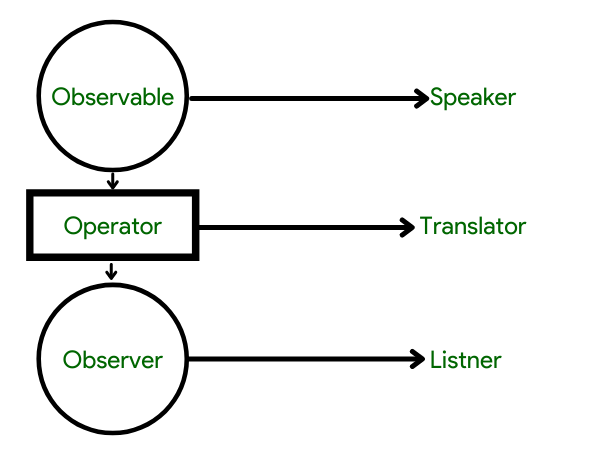
RxJava is a library for composing asynchronous and event-based programs using observable sequences. It is part of the larger ReactiveX family, which includes implementations for several languages like JavaScript (RxJS) and Python (RxPy). At its core, RxJava leverages the Observer pattern, allowing objects to subscribe to and react to changes emitted by an Observable source.
1.1 The Asynchronous Challenge in Java
Traditionally, handling asynchronous operations in Java involved working with threads or using Future and CompletableFuture. While these are powerful tools, they can quickly lead to complex code when dealing with multiple streams of data or events.
Java's native concurrency mechanisms often involve manual synchronization and management of thread pools, which can introduce errors and make the code hard to maintain. RxJava steps in by abstracting these complexities, providing a declarative way to express asynchronous flows.
1.2 The Power of Reactive Programming
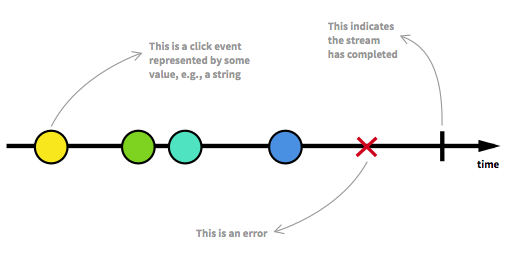
Reactive programming, the philosophy behind RxJava, is about building systems that respond to changes in real-time. It allows developers to express transformations and combinations of asynchronous data streams (or events) declaratively. Instead of manually orchestrating the flow of data and handling each event separately, reactive programming lets you compose complex pipelines using simple operators.
1.3 Core Concepts of RxJava
- Observable: An entity that emits a stream of data over time, either immediately or at some future point. The observable can emit multiple items, errors, or completion signals.
- Observer: An entity that subscribes to an observable to receive emissions and handle them (or handle errors/completion).
- Schedulers: RxJava provides built-in thread management, allowing developers to specify on which threads the observable should emit data and on which threads the observer should handle it.
- Operators: These are functions that allow you to transform, filter, or combine data streams emitted by observables.
Example: Basic Observable and Observer
import io.reactivex.Observable;
public class RxJavaExample {
public static void main(String[] args) {
Observable<String> observable = Observable.just("Hello", "RxJava", "World");
observable.subscribe(
item -> System.out.println("Received: " + item), // onNext
throwable -> System.err.println("Error: " + throwable), // onError
() -> System.out.println("Done!") // onComplete
);
}
}
In this simple example, we create an Observable that emits three items ("Hello", "RxJava", and "World"). The observer subscribes and prints each item as it is emitted, handling the completion and any potential errors.
2. Key Features of RxJava
Now that we understand what RxJava is and how it works at a basic level, let’s explore some of its key features that make it indispensable for modern Java applications.
2.1 Concurrency Management with Schedulers
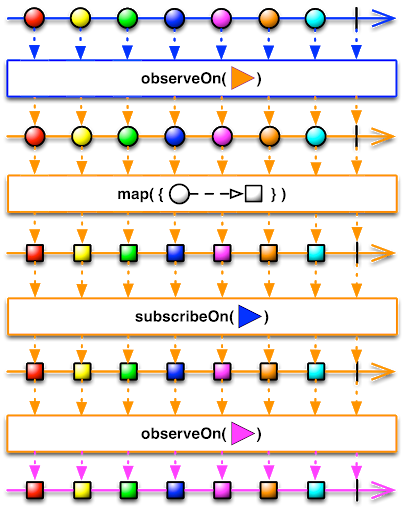
Schedulers are one of RxJava's standout features. With RxJava, you can effortlessly move work between threads without worrying about synchronization or managing thread pools manually. By specifying different schedulers, you can control where your observable executes and where the observer handles results.
Example: Using Schedulers to Manage Concurrency
import io.reactivex.Observable;
import io.reactivex.schedulers.Schedulers;
public class RxJavaSchedulerExample {
public static void main(String[] args) {
Observable<String> observable = Observable.just("Hello", "from", "RxJava");
observable
.subscribeOn(Schedulers.io()) // run emission on IO thread
.observeOn(Schedulers.computation()) // handle results on computation thread
.subscribe(item -> System.out.println("Received: " + item + " on " + Thread.currentThread().getName()));
}
}
In this example, the observable is set to emit items on the IO thread (ideal for tasks like network calls or disk reads), while the observer processes the data on the computation thread (suited for CPU-bound tasks).
2.2 Error Handling in RxJava
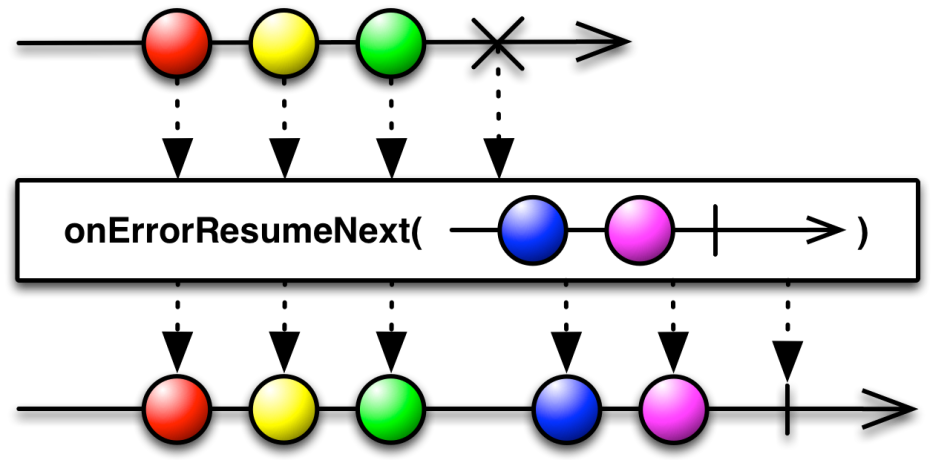
Error handling is critical in asynchronous programming, as exceptions can occur at various stages in the pipeline. RxJava provides multiple operators for error handling, such as onErrorReturn, onErrorResumeNext, and retry.
Example: Error Handling with onErrorReturn
import io.reactivex.Observable;
public class RxJavaErrorHandlingExample {
public static void main(String[] args) {
Observable<Integer> observable = Observable.create(emitter -> {
emitter.onNext(1);
emitter.onNext(2);
emitter.onError(new RuntimeException("An error occurred!"));
});
observable
.onErrorReturn(throwable -> -1) // handle error by returning a fallback value
.subscribe(item -> System.out.println("Received: " + item),
throwable -> System.err.println("Error: " + throwable),
() -> System.out.println("Done"));
}
}
In this case, when an error occurs during emission, onErrorReturn provides a fallback value, allowing the flow to continue without crashing the application.
3. Real-World Use Cases of RxJava
RxJava shines in applications that deal with a lot of asynchronous data, such as network requests, real-time UI updates, or handling large streams of data.
3.1 Using RxJava for API Calls
Consider an example where you need to make multiple API calls and combine their results. Without RxJava, this would typically involve callback hell or complex Future chaining. With RxJava, you can compose these operations declaratively.
Example: Making Concurrent API Calls
import io.reactivex.Observable;
import io.reactivex.schedulers.Schedulers;
public class ApiCallExample {
public static void main(String[] args) {
Observable<String> apiCall1 = Observable.just("Result from API 1").subscribeOn(Schedulers.io());
Observable<String> apiCall2 = Observable.just("Result from API 2").subscribeOn(Schedulers.io());
Observable.zip(apiCall1, apiCall2, (result1, result2) -> result1 + " & " + result2)
.subscribe(System.out::println);
}
}
In this example, two API calls run concurrently on the IO scheduler. The results are combined using the zip operator, and the final result is printed.
3.2 Combining UI Events in Android
RxJava has extensive use in Android for handling UI events. Imagine needing to wait for a user to fill out multiple fields before enabling a button. With RxJava, you can easily combine these streams.
Example: Combining Form Input Events
import io.reactivex.Observable;
import io.reactivex.functions.Function3;
public class FormValidationExample {
public static void main(String[] args) {
Observable<Boolean> nameFilled = Observable.just(true); // Simulating user input
Observable<Boolean> emailFilled = Observable.just(true);
Observable<Boolean> passwordFilled = Observable.just(true);
Observable.combineLatest(nameFilled, emailFilled, passwordFilled,
(Function3<Boolean, Boolean, Boolean, Boolean>) (name, email, password) -> name && email && password)
.subscribe(valid -> System.out.println("Form is valid: " + valid));
}
}
This example showcases how you can combine multiple input streams to determine when a form is valid.
4. Conclusion
RxJava brings an incredible level of power and simplicity to managing asynchronous operations in Java. By leveraging observables, observers, and a rich set of operators, RxJava enables you to build reactive, maintainable, and clean code. Whether it's handling API calls, managing user input, or controlling concurrency, RxJava can greatly enhance your application's responsiveness and performance.
If you have any questions about RxJava or want to explore more examples, feel free to leave a comment below!
Read more at : Secrets of RxJava: Why It’s the Key to Managing Asynchronous Operations in Java