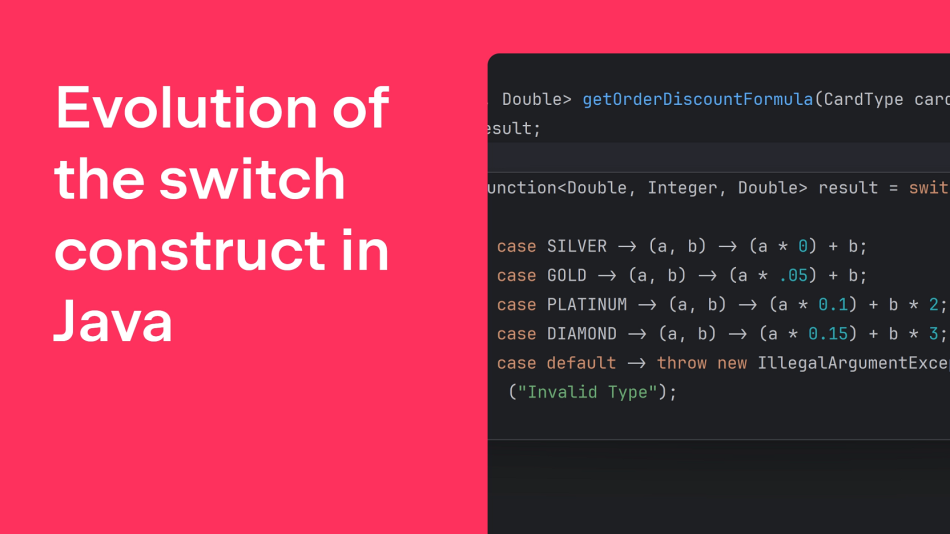
Switch Case in Java 17 and Java 21 for Cleaner Code
In every developer's journey, certain constructs become indispensable, and the switch statement is one of them. Java has long been associated with traditional switch-case structures, but with the release of Java 17 and Java 21, we witness a revol...
1. The Evolution of Switch Statements in Java
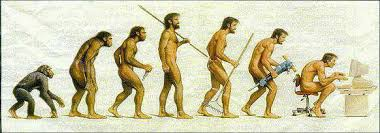
Since its inception, the switch statement in Java has been a go-to solution for managing conditional logic involving multiple outcomes. However, traditional switch statements often lacked flexibility, readability, and functionality compared to constructs like if-else.
1.1 Traditional Switch Case Pre-Java 17
Before Java 17, switch had a somewhat limited capability. It supported only primitive types, enum constants, and a few reference types. There were common pitfalls—such as forgetting to include the break statement—that could lead to logic errors. Below is an example of a typical switch statement:
public class TraditionalSwitch {
public static void main(String[] args) {
int day = 3;
String dayName;
switch (day) {
case 1:
dayName = "Monday";
break;
case 2:
dayName = "Tuesday";
break;
case 3:
dayName = "Wednesday";
break;
default:
dayName = "Invalid day";
}
System.out.println(dayName);
}
}
1.2 The Problems with Old Switch
The issues were obvious—verbose syntax, error-prone breaks, and the inability to handle more sophisticated control flows. If you forgot a break, unintended fall-through behavior occurred, which could lead to bugs.
2. Switch Case Enhancements in Java 17
With the release of Java 17, the switch-case functionality was significantly improved. Java 17 introduced pattern matching and switch expressions, allowing for more concise and flexible code.
2.1 Switch as an Expression
One of the key changes is the ability to use switch as an expression, returning values directly. This avoids multiple case blocks with redundant break statements and reduces potential for errors.
public class SwitchExpression {
public static void main(String[] args) {
int day = 3;
String dayName = switch (day) {
case 1 -> "Monday";
case 2 -> "Tuesday";
case 3 -> "Wednesday";
default -> "Invalid day";
};
System.out.println(dayName);
}
}
In this example, we see how Java 17’s switch expressions return values directly with less code. The arrow (->) operator replaces the need for both break and curly braces, making the code cleaner and easier to read.
2.2 Yield in Switch Expressions
When you need more complex logic within a case, you can use the yield keyword. This allows you to perform calculations or execute additional statements before returning the result.
public class YieldInSwitch {
public static void main(String[] args) {
int score = 85;
String grade = switch (score) {
case 90, 100 -> "A";
case 80, 89 -> "B";
case 70, 79 -> "C";
case 60, 69 -> "D";
default -> {
if (score < 60) {
yield "F";
} else {
yield "Invalid score";
}
}
};
System.out.println("Grade: " + grade);
}
}
Here, the yield keyword ensures that even in complex cases, we can return values seamlessly, avoiding clutter and confusion in our switch logic.
3. Switch Case in Java 21: Enhancing Pattern Matching
Java 21 builds on the foundation laid by Java 17 and further enhances the switch statement, particularly in pattern matching and more refined control over logic.
3.1 Pattern Matching with Switch
One of the standout features in Java 21 is enhanced pattern matching in switch cases. This feature allows switch to match against types, making it possible to deconstruct objects directly within cases.
public class PatternMatchingSwitch {
public static void main(String[] args) {
Object obj = "Hello, Java!";
String result = switch (obj) {
case String s -> "It's a string: " + s;
case Integer i -> "It's an integer: " + i;
default -> "Unknown type";
};
System.out.println(result);
}
}
Java 21 introduces a more flexible switch by allowing cases to match against object types, reducing the need for multiple instanceof checks and casting operations.
3.2 Exhaustiveness in Pattern Matching
Java 21 further introduces exhaustiveness in pattern matching, ensuring that all potential cases are covered. If the switch is not exhaustive, the compiler will raise an error, prompting the developer to cover all possible scenarios.
public class Exhaustiveness {
sealed interface Shape permits Circle, Rectangle {}
record Circle(double radius) implements Shape {}
record Rectangle(double length, double width) implements Shape {}
public static void main(String[] args) {
Shape shape = new Circle(3.0);
String result = switch (shape) {
case Circle c -> "Circle with radius " + c.radius();
case Rectangle r -> "Rectangle with length " + r.length() + " and width " + r.width();
};
System.out.println(result);
}
}
Here, Java 21 ensures that every possible case for a sealed class is covered, preventing errors at runtime due to unhandled cases.
4. Why Use Java 17 and Java 21 for Switch Statements?
The improvements introduced in Java 17 and Java 21 make the switch statement a far more powerful tool for developers. The concise syntax, ability to handle types, and elimination of common pitfalls like missing break statements make these updates invaluable. By adopting these newer versions, you can reduce boilerplate, enhance code readability, and minimize errors.
4.1 Cleaner Code
Both Java 17 and Java 21 emphasize conciseness and readability. As demonstrated in the examples above, you can achieve the same logic with fewer lines of code, making it easier to maintain and understand.
4.2 Better Control over Complex Logic
With yield and pattern matching, Java 17 and Java 21 provide better mechanisms to handle complex conditions within switch cases. This makes the switch structure applicable in a broader range of scenarios than ever before.
4.3 Enhanced Type-Safety
Switches in Java 21 offer type-safety improvements with pattern matching, allowing you to catch type mismatches at compile-time rather than runtime.
5. Conclusion
The switch statement has evolved tremendously in Java 17 and Java 21, transforming it from a basic control structure into a powerful and flexible tool. By adopting these modern features, you not only make your code cleaner and more efficient but also reduce the chances of errors and improve maintainability. Whether you are working on a simple conditional statement or handling complex object structures, these versions of Java offer the secrets to mastering control flow.
Have questions about switch cases in Java 17 or Java 21? Drop them in the comments below, and let's dive into the discussion!
Read more at : Switch Case in Java 17 and Java 21 for Cleaner Code