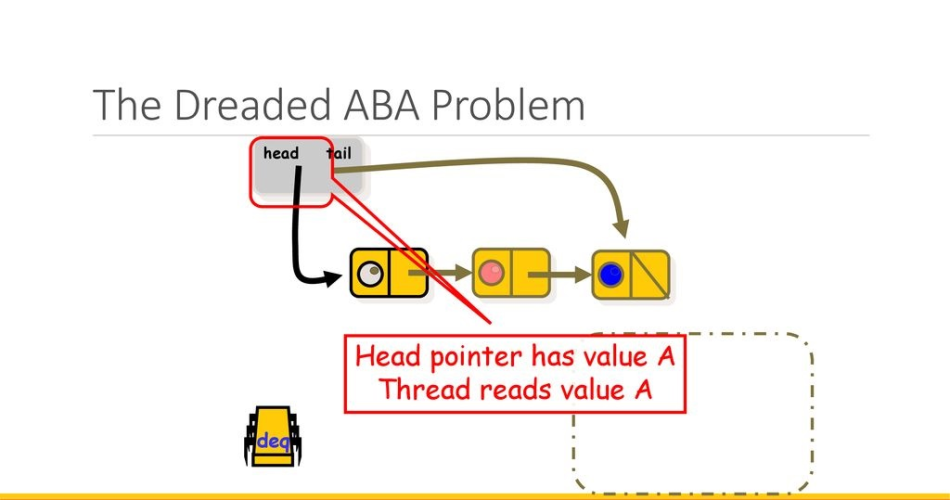
Techniques for Handling ABA Problems in CAS with Java
Concurrency is a vital aspect of modern software development, but it can introduce complex issues such as the ABA problem in Compare-And-Swap (CAS) operations. Understanding these concepts and how to address them effectively is crucial for buildi...
1. Understanding CAS and the ABA Problem
1.1 What is CAS (Compare-And-Swap)?
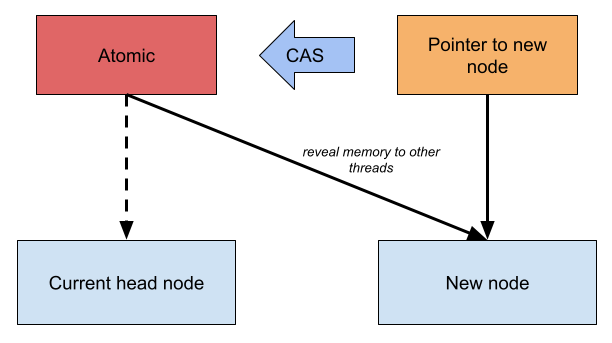
Compare-And-Swap (CAS) is a fundamental atomic operation used in concurrent programming to achieve synchronization. It works by comparing the current value of a variable with a specified value, and if they are equal, it updates the variable to a new value. This operation is atomic, meaning it is executed as a single, indivisible unit, preventing other threads from interfering.
Example Code: CAS Operation in Java
import java.util.concurrent.atomic.AtomicInteger;
public class CASExample {
private final AtomicInteger value = new AtomicInteger(0);
public boolean compareAndSet(int expectedValue, int newValue) {
return value.compareAndSet(expectedValue, newValue);
}
public static void main(String[] args) {
CASExample example = new CASExample();
System.out.println("Initial Value: " + example.value.get());
boolean result = example.compareAndSet(0, 1);
System.out.println("Update Successful: " + result);
System.out.println("New Value: " + example.value.get());
}
}
In this example, compareAndSet method of AtomicInteger is used to perform a CAS operation.
1.2 What is the ABA Problem?
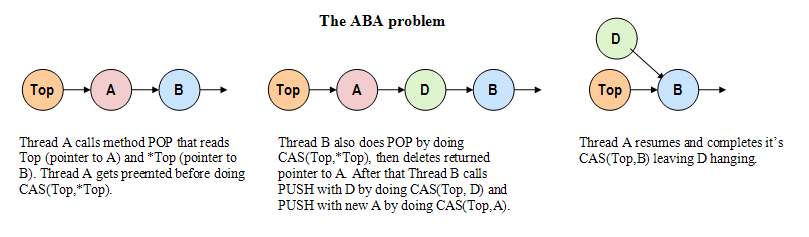
The ABA problem occurs in concurrent systems when a thread reads a value, observes no changes, but the value has actually changed and then reverted back to its original state. In CAS operations, this can lead to incorrect assumptions that the value has not changed when, in reality, it has.
Example of ABA Problem
Consider a scenario where a variable's value changes from A to B and then back to A. A thread might incorrectly assume that the value has not changed during its operation.
2. Techniques to Resolve the ABA Problem
2.1 Using Versioning
One effective way to address the ABA problem is by adding versioning to the CAS operation. Instead of storing just the value, you also store a version number. Each time the value is updated, the version number is incremented. This way, you can ensure that not only the value but also the version is checked, preventing the ABA problem.
Example Code: Versioned CAS
import java.util.concurrent.atomic.AtomicInteger;
public class VersionedCASExample {
private static class VersionedValue {
final int value;
final int version;
VersionedValue(int value, int version) {
this.value = value;
this.version = version;
}
}
private final AtomicInteger versionedValue = new AtomicInteger(new VersionedValue(0, 0));
public boolean compareAndSet(int expectedValue, int newValue, int expectedVersion) {
VersionedValue current = versionedValue.get();
return current.value == expectedValue && current.version == expectedVersion &&
versionedValue.compareAndSet(current, new VersionedValue(newValue, expectedVersion + 1));
}
public static void main(String[] args) {
VersionedCASExample example = new VersionedCASExample();
System.out.println("Initial Value: " + example.versionedValue.get().value);
boolean result = example.compareAndSet(0, 1, 0);
System.out.println("Update Successful: " + result);
System.out.println("New Value: " + example.versionedValue.get().value);
}
}
In this code, VersionedValue class encapsulates both the value and version, ensuring that the CAS operation considers both during updates.
2.2 Using a More Complex Data Structure
Another approach is to use more advanced data structures designed to handle concurrency issues, such as concurrent linked lists or queues. These structures are designed with built-in mechanisms to avoid the ABA problem and other concurrency issues.
Example Code: Concurrent Data Structure
import java.util.concurrent.ConcurrentLinkedQueue;
public class ConcurrentQueueExample {
private final ConcurrentLinkedQueue<Integer> queue = new ConcurrentLinkedQueue<>();
public void addElement(int element) {
queue.add(element);
}
public Integer removeElement() {
return queue.poll();
}
public static void main(String[] args) {
ConcurrentQueueExample example = new ConcurrentQueueExample();
example.addElement(1);
example.addElement(2);
System.out.println("Removed Element: " + example.removeElement());
}
}
In this example, ConcurrentLinkedQueue is used to manage concurrent access to the queue, thus reducing the likelihood of encountering ABA problems.
3. Conclusion
The ABA problem in CAS operations is a subtle but significant issue in concurrent programming. By understanding and implementing techniques such as versioning or using advanced concurrent data structures, you can effectively mitigate this problem. If you have any questions or need further clarification on handling the ABA problem or other concurrency issues, feel free to comment below!
Read more at : Techniques for Handling ABA Problems in CAS with Java