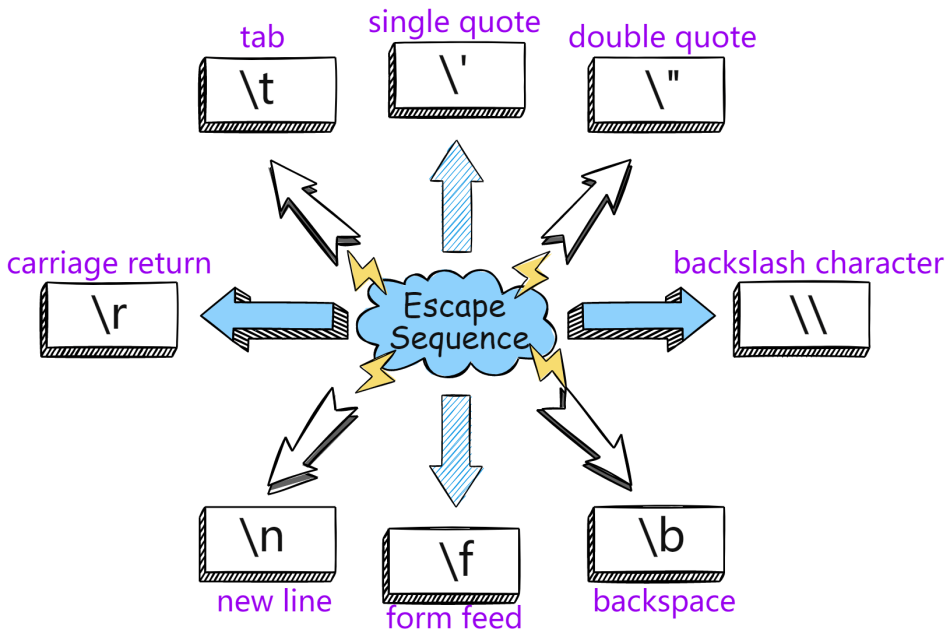
Escaping Strings in Java: How to Handle Special Characters Effectively
Handling special characters in Java can be tricky for developers, especially when it comes to working with strings that involve escape sequences. This might seem like a small issue, but managing it incorrectly can lead to serious bugs, security v...
1. What Does Escaping a String Mean in Java?
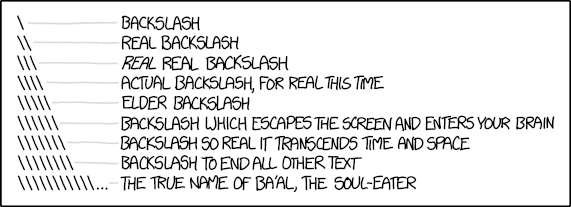
Escaping a string refers to the process of prefixing certain characters with a backslash () so that they are interpreted in a specific way by the Java compiler. Special characters, such as newline ( ), tab ( ), or double quotes ("), have specific meanings in Java, and escaping them ensures that they are handled correctly by the program.
1.1 Why Is Escaping Important?
If a program encounters an unescaped special character, it may produce unintended behavior. For instance, forgetting to escape double quotes in a string might cause the compiler to mistake it for the end of the string, leading to syntax errors. On a deeper level, properly escaping strings is essential for avoiding injection attacks, especially in SQL queries and HTML content.
1.2 How Does Java Handle Escape Sequences?
Java provides several built-in escape sequences that help manage common scenarios. Here are some of the most frequently used escape characters in Java:
- : Newline
- : Tab
- \: Backslash
- ": Double quote
- ': Single quote
Let’s see how these are used in practice.
1.3 Code Example: Using Escape Sequences in Java Strings
public class EscapeStringExample {
public static void main(String[] args) {
String text = "Java says: "Hello, World!" New Line Tabbed space";
System.out.println(text);
}
}
In this example, the string includes double quotes around "Hello, World!", a newline ( ) to move to the next line, and a tab ( ) for indented text.
1.4 Demo Results
When you run this code, the output looks like this:
Java says: "Hello, World!"
New Line Tabbed space
As you can see, the double quotes are correctly displayed within the string, the new line is inserted, and the tab creates a proper indentation. Without these escape sequences, the program would fail to execute or produce incorrect results.
2. Escaping Strings Dynamically
What if you need to escape a string dynamically, such as receiving user input containing special characters? Java’s built-in methods can help ensure that these characters are escaped correctly.
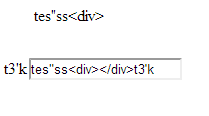
2.1 Escaping Backslashes Dynamically
Backslashes are especially tricky since they are used as the escape character itself. If you want to include a literal backslash in a string, you must escape it with another backslash.
Example:
public class EscapeBackslash {
public static void main(String[] args) {
String path = "C:\Program Files\Java";
System.out.println(path);
}
}
In this example, the backslashes in the file path are escaped so that they are displayed as intended.
2.2 Handling Escape Sequences in User Input
Imagine you're developing a program where users can input text that may include special characters like quotes or backslashes. You want to ensure that your program can handle this input without breaking. Let’s look at how you can sanitize and escape user input in Java.
public class EscapeUserInput {
public static String escapeSpecialChars(String input) {
return input.replace("\", "\").replace(""", "\"");
}
public static void main(String[] args) {
String userInput = "He said, "Escape this!"";
String escapedInput = escapeSpecialChars(userInput);
System.out.println("Escaped input: " + escapedInput);
}
}
The output will be:
Escaped input: He said, "Escape this!"
This demonstrates how you can escape user-provided text, ensuring that special characters are handled safely and correctly. In real-world scenarios, this approach is critical for preventing code injection attacks and other security issues.
3. Escaping Strings in SQL Queries
One of the most common and dangerous mistakes developers make is neglecting to properly escape strings in SQL queries. This can leave applications vulnerable to SQL injection attacks. Java's PreparedStatement helps by escaping inputs for you, but you must still understand how to handle strings properly.
3.1 Example of Escaping Strings in SQL
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.SQLException;
public class SqlEscapeExample {
public static void main(String[] args) {
String userInput = "O'Reilly"; // Apostrophe can break SQL query
String query = "SELECT * FROM users WHERE name = ?";
try (Connection con = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydb", "user", "password")) {
PreparedStatement ps = con.prepareStatement(query);
ps.setString(1, userInput); // Escape handled by PreparedStatement
ps.executeQuery();
} catch (SQLException e) {
e.printStackTrace();
}
}
}
In this example, the apostrophe in the user’s input (O'Reilly) would normally cause an SQL syntax error. However, by using a PreparedStatement, Java automatically escapes the string to prevent the query from failing.
The program executes without issues, even though the input contains an apostrophe. This method helps prevent SQL injection vulnerabilities and ensures that special characters in user input do not break your queries.
4. Best Practices for Escaping Strings in Java
Escaping strings is more than just avoiding syntax errors—it's a critical step for writing secure and reliable Java applications. Here are some best practices to keep in mind:
Always Escape User Input
When receiving input from users, make sure to escape special characters to prevent errors and potential security risks.
Use Java's Built-In Tools
Whenever possible, rely on Java’s built-in mechanisms, such as PreparedStatement for SQL queries and escape sequences for strings, to handle special characters.
Be Aware of Special Characters in External Data
If your program handles data from external sources, such as files, databases, or APIs, ensure that special characters are properly escaped to avoid parsing issues or security vulnerabilities.
5. Conclusion
Handling special characters in Java requires careful attention, especially when dealing with user input or external data sources. By understanding how to escape strings properly, you can write more reliable, secure, and maintainable code. Remember to always use Java's built-in tools for escaping, particularly in sensitive areas like SQL queries.
Have any questions or suggestions? Feel free to comment below, and we’ll be happy to help!
Read more at : Escaping Strings in Java: How to Handle Special Characters Effectively