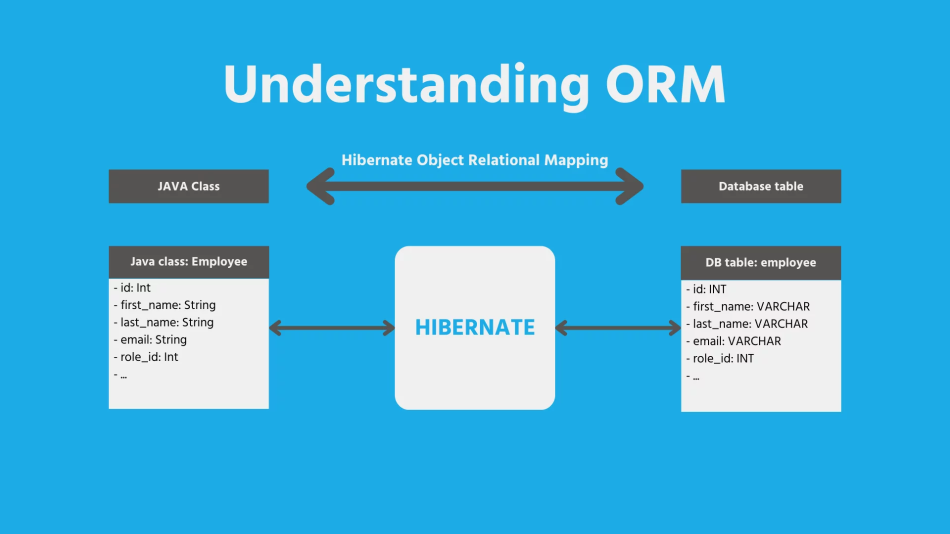
How Hibernate ORM Works Under the Hood
Hibernate ORM (Object-Relational Mapping) is a popular framework in the Java ecosystem that simplifies database interactions by mapping Java objects to database tables. But what really happens behind the scenes? In this article, we'll dive deep i...
1. Overview of Hibernate ORM
Hibernate ORM acts as a bridge between your Java application and the database, abstracting away the complexities of data management. By mapping Java classes to database tables, Hibernate handles CRUD (Create, Read, Update, Delete) operations with ease.
1.1 How Hibernate Maps Java Objects to Database Tables
At its core, Hibernate uses metadata annotations or XML configuration to define how Java classes are mapped to database tables. Each class represents a table, and each field in the class corresponds to a column in the table. For example:
@Entity
@Table(name = "users")
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@Column(name = "username")
private String username;
@Column(name = "email")
private String email;
// Getters and setters
}
Hibernate provides two primary ways to define mappings between Java objects and database tables:
- Annotations: You can use annotations directly in your Java classes to define how they should be mapped to database tables. For example, @Entity denotes a class as an entity that should be mapped to a table, and @Table specifies the table name. Properties in the class can be annotated with @Column to map to specific columns in the table.
- XML Configuration: Alternatively, Hibernate allows you to define mappings in XML configuration files. These files specify how each class maps to a table and how each property maps to a column.
Hibernate uses configuration files (e.g., hibernate.cfg.xml) to set up database connections, specify dialects, and define other properties. The configuration file tells Hibernate how to connect to the database and what the underlying database specifics are.
1.2 The Hibernate Session
A Session is used to interact with the database. It provides methods for CRUD operations (create, read, update, delete) and manages the persistence state of objects.
Hibernate uses a SessionFactory to create Session instances. It’s a thread-safe object that is responsible for creating sessions and managing cache and transactions.
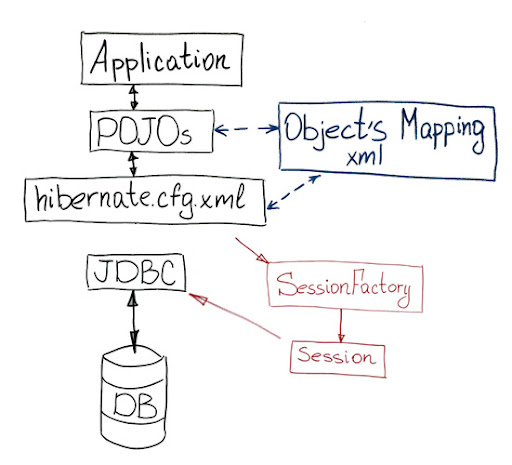
Here’s how Session works:
- Open Session: Hibernate opens a session to interact with the database.
- Transaction Management: Sessions are used within transactions to ensure atomicity.
- Session Operations: CRUD operations are performed using the session.
- Close Session: After operations are complete, the session is closed.
2. How Hibernate Handles Transactions
Transactions ensure that a series of operations either complete successfully or fail as a unit. Hibernate abstracts transaction management, allowing you to work with transactions in a more intuitive way.
Transactions in Hibernate are managed by the Transaction interface, which allows you to begin, commit, or rollback transactions. Here’s how it works:
- Begin Transaction: Hibernate's Session object is used. You begin a transaction with the beginTransaction() method on the Session object. This method returns a Transaction object, which represents the transactional context.
- Commit Transaction: To save the changes made during the transaction to the database, you call the commit() method on the Transaction object. This method ensures that all the changes made during the transaction are persisted.
- Rollback Transaction: If an error occurs during the transaction, you can call the rollback() method to undo all changes made during the transaction. This method reverts the database to its state before the transaction began.
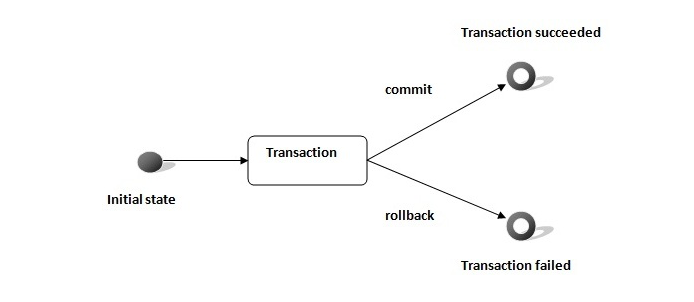
A Session maintains a persistence context, which tracks changes to entities and manages their synchronization with the database.
- Auto-Flush: Hibernate’s Session automatically flushes changes to the database when a transaction is committed. This means that any pending changes are sent to the database, ensuring consistency between the session and the database.
- Transaction Synchronization: Hibernate synchronizes its transactions with the underlying database using the Java Transaction API (JTA) or JDBC transactions. This synchronization ensures that the transaction boundaries are properly managed and that changes are committed or rolled back as a single unit of work.
For applications that require distributed transactions or need to integrate with Java EE environments, Hibernate can be configured to use the Java Transaction API (JTA). JTA allows for managing transactions across multiple resources, such as databases and messaging systems.
3. Hibernate's Internal Mechanisms
3.1 Bytecode Enhancement
Bytecode enhancement is a technique used by Hibernate to optimize the performance of entity operations. It involves modifying the bytecode of Java classes at runtime, enabling more efficient operations and reducing overhead.
How Bytecode Enhancement Works
Instrumentation: Hibernate uses bytecode instrumentation to modify the compiled bytecode of entity classes. This modification happens at runtime, allowing Hibernate to inject additional logic into the entity classes without altering the original source code. This process is typically done using tools like Java agents or bytecode manipulation libraries such as ASM or Javassist.
Enhancement Capabilities:
- Lazy Loading: Enhancement allows for the lazy loading of entity properties. Instead of loading all properties immediately, Hibernate can delay the loading of specific fields until they are actually accessed. This helps in reducing the initial load time and memory usage.
- Dirty Checking: Hibernate can enhance entities to support efficient dirty checking. This means it can keep track of changes to entities more effectively, only persisting modified fields to the database rather than the entire entity.
- Field Access: Bytecode enhancement can optimize access to private fields of entities, allowing Hibernate to directly interact with fields instead of relying on getters and setters. This reduces the overhead associated with property access.
Enhancement can be applied at build time using tools integrated into the build process, such as Maven or Gradle plugins. Some enhancement operations are performed at runtime, often using Java agents or specific Hibernate configurations.
Benefits of Bytecode Enhancement
By optimizing entity operations, bytecode enhancement can significantly improve performance, particularly in scenarios with complex entity relationships or large datasets.
Lazy loading and field access optimizations reduce the amount of data loaded into memory and the frequency of database queries, which leads to more efficient resource usage.
3.2 Proxy Objects
Proxy objects are another critical mechanism used by Hibernate to optimize performance, specifically through lazy loading.
How Proxy Objects Work
Lazy Loading: Hibernate uses proxy objects to implement lazy loading for associations and entities. When you fetch an entity with associations that are marked as lazy, Hibernate does not load the associated data immediately. Instead, it returns a proxy object.
Proxy Object Characteristics:
- Placeholder: A proxy object acts as a placeholder for the actual entity. It contains a reference to the entity’s identifier and the session it belongs to.
- On-Demand Loading: The actual data is loaded from the database only when the properties or methods of the proxy are accessed. This is achieved through method interception.
- Transparent Access: From the developer’s perspective, interacting with a proxy object is similar to interacting with a fully-loaded entity. Hibernate handles the behind-the-scenes data retrieval.
How Proxies Are Created
Dynamic Proxies: Hibernate generates dynamic proxies at runtime. These proxies extend the entity class and implement the entity’s interfaces, allowing them to intercept method calls and load data as needed.
Static Proxies: In some cases, static proxies can be used, especially when bytecode enhancement is employed. These proxies are pre-generated and provide the same lazy-loading benefits.
Benefits of Proxy Objects
Proxy objects enable efficient data loading by fetching related data only when it is actually required. This reduces the amount of data loaded into memory and minimizes unnecessary database queries.
By deferring data loading until it is needed, proxy objects help improve overall application performance, especially in scenarios involving complex relationships or large datasets.
4. Conclusion
Hibernate ORM is a powerful tool for managing database interactions in Java applications. By understanding its internal mechanisms, such as how it maps objects, handles transactions, and optimizes performance, you can leverage its full potential in your projects.
If you have any questions or need further clarification, feel free to leave a comment below!
Read more at : How Hibernate ORM Works Under the Hood