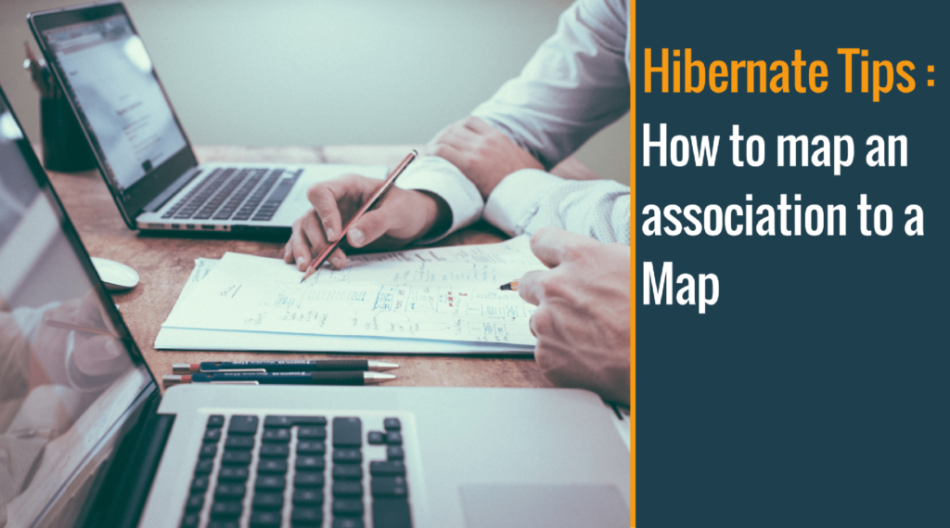
Mastering the @MapKey Annotation for Map Relationships
When working with Hibernate, managing relationships between entities efficiently is crucial for maintaining performance and clarity in your application. One powerful tool for this purpose is the @MapKey annotation, which provides a way to handle ...
1. Understanding the @MapKey Annotation
The @MapKey annotation is used in Hibernate to define a map relationship where each entry in the map is a key-value pair. This allows you to store associations between entities using a map rather than a list, which can be beneficial in scenarios where you need to access elements by a unique key.
1.1 What is @MapKey?
The @MapKey annotation specifies the property of the map's key when the map is used in an entity. This is particularly useful when you want to use a non-primary key field as the map's key.
I have another post about Map that you might find interesting. Feel free to check it out!
For example, consider an Employee entity where you want to map employees to their unique email addresses:
@Entity
public class Employee {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
@ElementCollection
@MapKeyColumn(name = "email")
@Column(name = "phone_number")
private Map<String, String> emailToPhoneNumberMap = new HashMap<>();
// Getters and setters
}
Here, @MapKeyColumn(name = "email") indicates that the email field is used as the key in the map, while phone_number is the value.
1.2 Why Use @MapKey?
Using @MapKey can simplify the management of collections where quick lookups by a unique attribute are needed. Instead of iterating through a list to find a specific item, a map allows direct access to elements based on the key, which can improve performance and reduce complexity in your code.
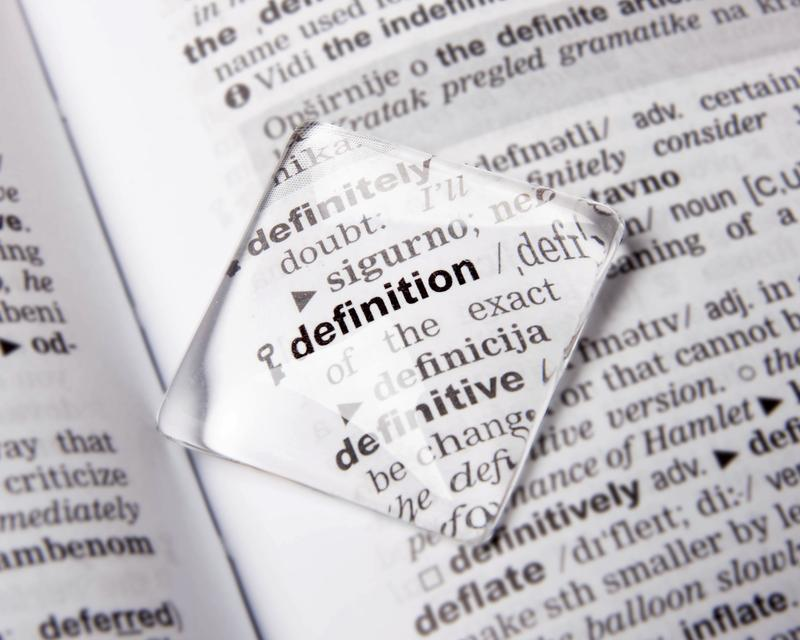
2. Implementing @MapKey in Hibernate
To see @MapKey in action, we'll walk through a complete example of setting up and using map relationships with Hibernate.
2.1 Defining the Entities
Let's define a Student entity and a Course entity where a student can enroll in multiple courses, and each course is uniquely identified by its code:
@Entity
public class Student {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
@ElementCollection
@MapKeyColumn(name = "course_code")
@Column(name = "grade")
private Map<String, String> courseGrades = new HashMap<>();
// Getters and setters
}
@Entity
public class Course {
@Id
private String courseCode;
private String courseName;
// Getters and setters
}
In this setup, course_code is the key for the courseGrades map, and each entry represents a course and the student's grade in that course.
2.2 Persisting and Querying Data
To persist and query data using these entities, you can use Hibernate's EntityManager or a repository pattern. Here’s an example of how to add a course and assign a grade to a student:
java
java
EntityManager em = entityManagerFactory.createEntityManager();
em.getTransaction().begin();
// Create a course
Course course = new Course();
course.setCourseCode("CS101");
course.setCourseName("Introduction to Computer Science");
em.persist(course);
// Create a student and assign a grade
Student student = new Student();
student.setName("John Doe");
student.getCourseGrades().put("CS101", "A");
em.persist(student);
em.getTransaction().commit();
em.close();
To query the grades of a student:
EntityManager em = entityManagerFactory.createEntityManager();
Student student = em.find(Student.class, 1L);
Map<String, String> grades = student.getCourseGrades();
grades.forEach((courseCode, grade) -> {
System.out.println("Course: " + courseCode + ", Grade: " + grade);
});
em.close();
3. Advantages of Using @MapKey
3.1 Efficient Lookups
Maps provide constant-time complexity for lookups, which is beneficial when you need to access elements quickly by a unique key. This can significantly improve performance compared to searching through a list.
3.2 Simplified Data Management
Managing relationships with maps can reduce the complexity of your code. Instead of handling separate lists and performing manual lookups, maps streamline the process of associating entities.
4. Conclusion
Optimizing Hibernate with the @MapKey annotation for map relationships can greatly enhance the efficiency and maintainability of your application. By understanding how to define and use map relationships effectively, you can improve performance and simplify your codebase.
If you have any questions or need further clarification, feel free to leave a comment below!
Read more at : Mastering the @MapKey Annotation for Map Relationships