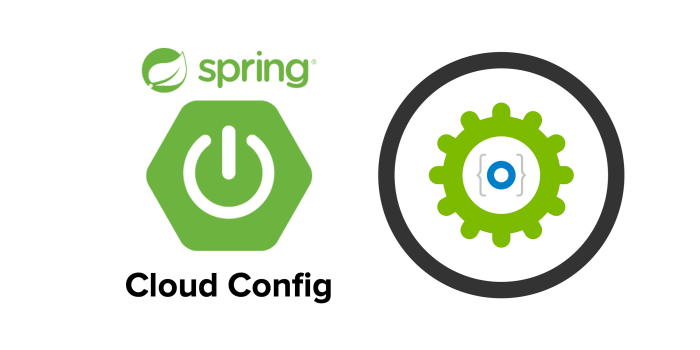
Spring Cloud Config with Managing Application Configuration
Managing configuration in modern distributed systems can be challenging. As applications scale and evolve, maintaining a centralized and consistent configuration across multiple environments is critical. Spring Cloud Config offers a robust soluti...
1. What is Spring Cloud Config?
Spring Cloud Config provides server-side and client-side support for externalized configuration in a distributed system. With Spring Cloud Config, you can store your configuration files in a remote repository (e.g., Git, SVN) and access them from multiple applications and environments.
Key Features:
+ Centralized configuration management
+ Dynamic property changes without redeploying applications
+ Support for various backend repositories (Git, SVN, native file system)
+ Encryption and decryption of sensitive properties
+ Centralized configuration management
+ Dynamic property changes without redeploying applications
+ Support for various backend repositories (Git, SVN, native file system)
+ Encryption and decryption of sensitive properties
2. Setting Up Spring Cloud Config Server
The Spring Cloud Config Server acts as a configuration management service that provides configuration properties to client applications. Let's start by creating a Config Server.
Step 1: Create a New Spring Boot Application
Create a new Spring Boot application with the following dependencies:
+ Spring Cloud Config Server
+ Spring Boot DevTools
+ Spring Web
+ Spring Cloud Config Server
+ Spring Boot DevTools
+ Spring Web
Maven Configuration (pom.xml):
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-config-server</artifactId>
</dependency>
</dependencies>
<dependencyManagement>
<dependencies>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-dependencies</artifactId>
<version>Hoxton.SR12</version>
<type>pom</type>
<scope>import</scope>
</dependency>
</dependencies>
</dependencyManagement>
Step 2: Enable the Config Server
In the main application class, annotate with @EnableConfigServer to enable the configuration server.
Main Application Class (ConfigServerApplication.java):
package com.example.configserver;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.cloud.config.server.EnableConfigServer;
@SpringBootApplication
@EnableConfigServer
public class ConfigServerApplication {
public static void main(String[] args) {
SpringApplication.run(ConfigServerApplication.class, args);
}
}
Step 3: Configure the Config Server
In the application.yml file, configure the Git repository that the Config Server will use to fetch configuration properties.
application.yml
server:
port: 8888
spring:
cloud:
config:
server:
git:
uri: github.com/your-username/config-repo
clone-on-start: true
Here, replace github.com/your-username/config-repo with your own Git repository URL containing configuration files.
3. Creating Configuration Files in the Git Repository
In your Git repository, create a new configuration file named application.yml or environment-specific files like application-dev.yml, application-prod.yml.
Example Configuration (application.yml):
server:
port: 8080
message:
greeting: "Hello, World!"
4. Setting Up Spring Cloud Config Client
Now, let's create a Spring Boot client application that retrieves its configuration from the Config Server.
Step 1: Create a New Spring Boot Application
Create another Spring Boot application with the following dependencies:
+ Spring Cloud Config Client
+ Spring Boot DevTools
+ Spring Web
+ Spring Cloud Config Client
+ Spring Boot DevTools
+ Spring Web
Maven Configuration (pom.xml):
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-config</artifactId>
</dependency>
</dependencies>
Step 2: Configure the Config Client
Read more at : Spring Cloud Config with Managing Application Configuration