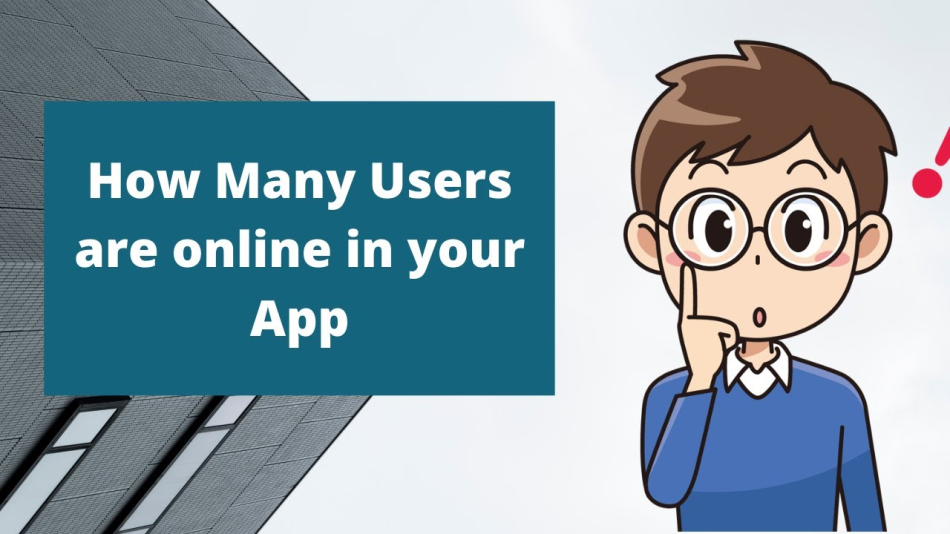
Strategies to Count the Number of Online Users in Your Application
The ability to track the number of online users in a web application is crucial for understanding user engagement, optimizing server performance, and even for security purposes. Whether you’re running a small blog or managing a large-scale platfo...
1. Understanding the Concept of "Online Users"
Before we dive into the technical details, it’s essential to clarify what "online" means. In web applications, an online user typically refers to someone who has an active session on the platform or has recently made an interaction with the server. This can be tracked through various mechanisms such as cookies, sessions, or websockets.
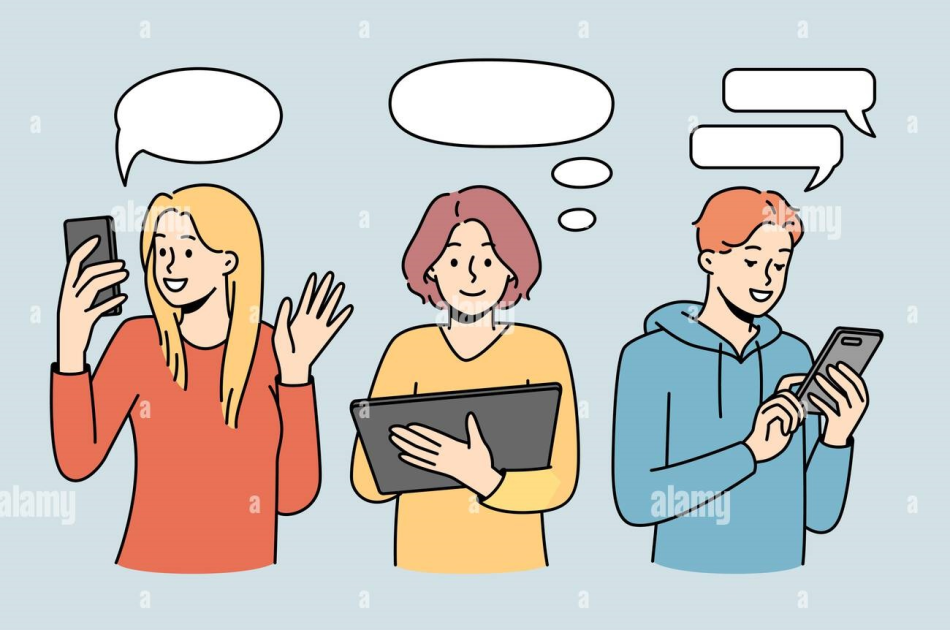
1.1 Defining "Online"
An "online" user in most web applications refers to someone who has recently interacted with the server, such as loading a page, making an API request, or performing an action. Tracking users who have performed an action within a certain timeframe (e.g., the last 5 minutes) is a common method of defining "online." In real-time systems, websockets can be used for instant communication, keeping the user status constantly updated.
1.2 Common Approaches to Track Online Users
There are several methods to count the number of online users. These include:
- Session-based tracking
- Token-based tracking (JWT)
- WebSocket tracking
- Redis or other in-memory databases for real-time updates
Each method has its pros and cons, which we’ll explore in the following sections.
2. Session-Based Tracking of Online Users
A popular and straightforward way to track online users is through sessions. When a user logs into the system or visits a website, a session is created. The server keeps track of this session, allowing you to count how many sessions are currently active.
2.1 Setting Up Session Management
In most web frameworks, session management is built-in. For example, in Java using Spring Boot, you can use HttpSession to manage sessions and keep track of users. Below is an example of how you can implement this:
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
import javax.servlet.http.HttpSession;
import java.util.concurrent.atomic.AtomicInteger;
@RestController
public class UserController {
private static final AtomicInteger onlineUsers = new AtomicInteger(0);
@GetMapping("/login")
public String login(HttpSession session) {
session.setAttribute("user", "loggedUser");
onlineUsers.incrementAndGet();
return "User logged in!";
}
@GetMapping("/logout")
public String logout(HttpSession session) {
session.invalidate();
onlineUsers.decrementAndGet();
return "User logged out!";
}
@GetMapping("/onlineUsers")
public String getOnlineUsers() {
return "Currently online users: " + onlineUsers.get();
}
}
2.2 How It Works
- When a user logs in, the /login endpoint is triggered, starting a new session. The session is stored in HttpSession.
- The AtomicInteger onlineUsers increments every time a new session is created.
- When the user logs out, the session is invalidated, and the onlineUsers count is decremented.
- The /onlineUsers endpoint gives you the current number of online users.
2.3 Demo Results
Once implemented, every time a user logs in, the online count will increment. Similarly, when they log out, it will decrement. A simple curl command or Postman can be used to test this, showing the number of active sessions.
$ curl localhost:8080/login
User logged in!
$ curl localhost:8080/onlineUsers
Currently online users: 1
$ curl localhost:8080/logout
User logged out!
$ curl localhost:8080/onlineUsers
Currently online users: 0
3. Using JWT Tokens for Tracking
Another method is using token-based authentication (like JWT - JSON Web Tokens). This method is stateless and highly scalable. You don’t need to manage sessions on the server side, as tokens can be validated independently.
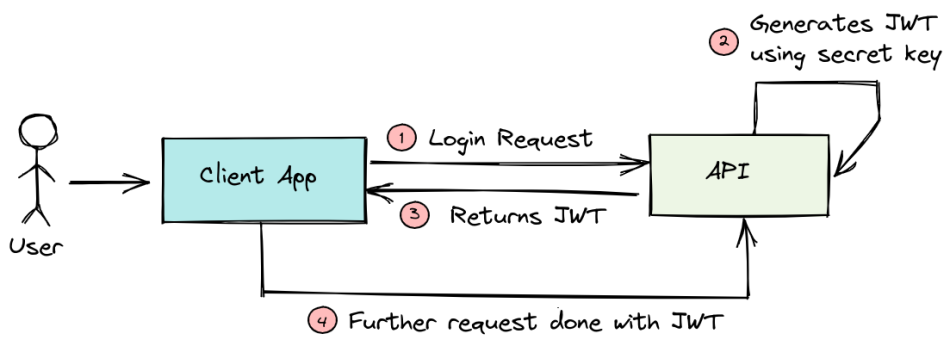
3.1 Setting Up JWT-Based User Tracking
You can track online users by verifying valid tokens and recording the number of tokens that are currently active. You could use a cache like Redis to store tokens and their expiration times, and then count the active tokens as a measure of online users.
Here’s an example in Spring Boot with Redis:
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.data.redis.core.RedisTemplate;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class TokenController {
@Autowired
private RedisTemplate<String, String> redisTemplate;
@GetMapping("/token")
public String createToken() {
String token = generateToken(); // JWT generation logic
redisTemplate.opsForValue().set(token, "active");
return "Token created and user is online!";
}
@GetMapping("/onlineTokens")
public Long getOnlineUsers() {
return redisTemplate.keys("*").stream().count();
}
}
3.2 How It Works
- The /token endpoint generates a new JWT token and stores it in Redis.
- The getOnlineUsers method checks how many active tokens are stored in Redis, which corresponds to the number of online users.
3.3 Demo Results
Each active token will represent an online user. When a token expires or is deleted, the user is no longer considered online. This method works well in distributed systems where scalability is a concern.
4. Real-Time Tracking with WebSockets
WebSockets provide real-time two-way communication between the server and clients, making them perfect for applications where you need to track online users in real-time, like in chat applications.
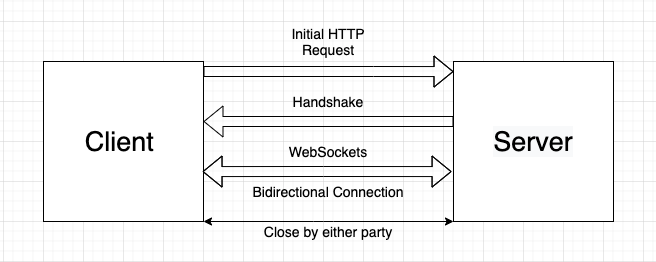
4.1 Implementing WebSocket-Based User Tracking
Here’s an example of how you can track users using WebSocket in Spring Boot:
import org.springframework.web.socket.TextMessage;
import org.springframework.web.socket.WebSocketSession;
import org.springframework.web.socket.handler.TextWebSocketHandler;
import java.util.concurrent.CopyOnWriteArrayList;
public class WebSocketUserHandler extends TextWebSocketHandler {
private static final CopyOnWriteArrayList<WebSocketSession> sessions = new CopyOnWriteArrayList<>();
@Override
public void afterConnectionEstablished(WebSocketSession session) throws Exception {
sessions.add(session);
session.sendMessage(new TextMessage("Welcome!"));
}
@Override
public void afterConnectionClosed(WebSocketSession session, CloseStatus status) throws Exception {
sessions.remove(session);
}
public int getOnlineUsersCount() {
return sessions.size();
}
}
4.2 How It Works
- When a user establishes a WebSocket connection, their session is stored in a CopyOnWriteArrayList.
- When the connection closes, the session is removed.
- The number of active WebSocket sessions corresponds to the number of online users.
5. Conclusion
Tracking the number of online users is an essential feature for any web application. Depending on the use case, you can choose between session-based, token-based, or real-time WebSocket tracking. Session-based tracking is ideal for traditional web apps, while token-based and WebSocket methods suit distributed systems and real-time applications, respectively.
If you have any questions or want to share your experience, feel free to comment below!
Read more at : Strategies to Count the Number of Online Users in Your Application