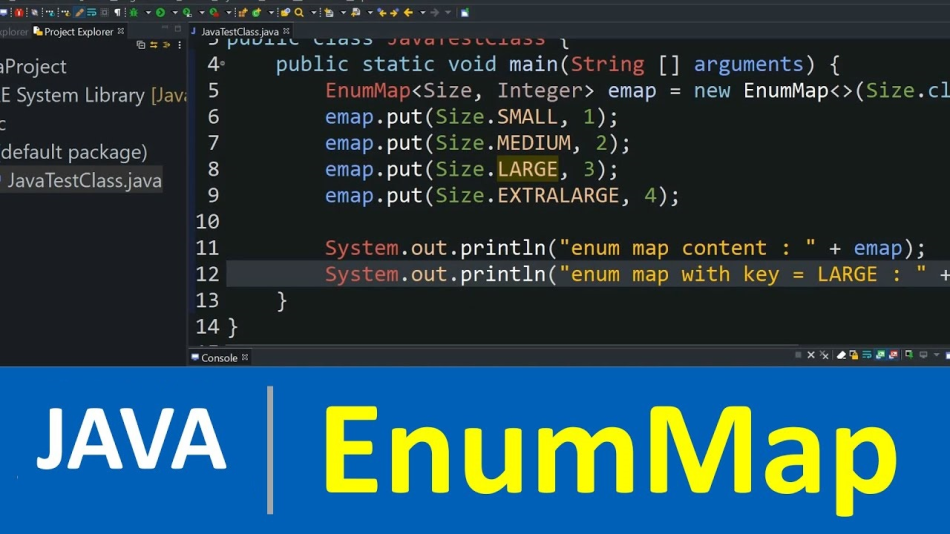
Understanding EnumMap in Java: Benefits, Usage, and Examples
Java collections offer a variety of powerful tools for managing groups of objects. One such tool, often overlooked, is the EnumMap. This specialized map is tailored for use with enum keys and provides both performance and functionality benefits. ...
1. Introduction to EnumMap
When dealing with enums in Java, the EnumMap provides an efficient and type-safe way to handle mappings where keys are restricted to a predefined set of constants. Unlike other map implementations, EnumMap is specifically designed for enums and offers performance improvements and enhanced readability.
1.1 What is EnumMap?
EnumMap is a specialized implementation of the Map interface that uses an array internally to store mappings from enum constants to values. This design makes EnumMap particularly fast and efficient when used with enums, leveraging the predictable and fixed nature of enum types.
import java.util.EnumMap;
enum Day {
MONDAY, TUESDAY, WEDNESDAY, THURSDAY, FRIDAY, SATURDAY, SUNDAY
}
public class EnumMapExample {
public static void main(String[] args) {
EnumMap<Day, String> schedule = new EnumMap<>(Day.class);
schedule.put(Day.MONDAY, "Work");
schedule.put(Day.SATURDAY, "Relax");
System.out.println(schedule);
}
}
In this example, EnumMap is used to map each day of the week to a string representing the activity for that day.
1.2 Why Use EnumMap?
The EnumMap is highly efficient because it uses a compact internal representation of the enum constants, which leads to faster access and reduced memory overhead compared to other map implementations. It's also inherently type-safe, as it ensures that only valid enum constants are used as keys.
1.3 Key Features of EnumMap
- Performance: EnumMap is faster than HashMap and TreeMap for enum keys due to its array-based internal storage.
- Memory Efficiency: It uses less memory than other map implementations since it only needs to store mappings for the enum constants.
- Type Safety: It ensures that only enum constants can be used as keys, preventing runtime errors.
2. How to Use EnumMap Effectively
Using EnumMap is straightforward, but there are best practices to follow to maximize its benefits. Below, we’ll explore how to create, initialize, and manipulate EnumMap instances effectively.
2.1 Creating and Initializing EnumMap
To create an EnumMap, you need to specify the enum type as the key type. Here's a more detailed example:
import java.util.EnumMap;
enum Season {
WINTER, SPRING, SUMMER, FALL
}
public class SeasonMapExample {
public static void main(String[] args) {
EnumMap<Season, String> seasonActivities = new EnumMap<>(Season.class);
seasonActivities.put(Season.WINTER, "Skiing");
seasonActivities.put(Season.SPRING, "Hiking");
seasonActivities.put(Season.SUMMER, "Swimming");
seasonActivities.put(Season.FALL, "Leaf Peeping");
System.out.println(seasonActivities);
}
}
In this example, each season is mapped to an activity typically associated with that time of year.
2.2 Manipulating EnumMap
EnumMap supports all standard map operations such as inserting, deleting, and querying entries. Here's how to perform some common operations:
import java.util.EnumMap;
enum Fruit {
APPLE, BANANA, ORANGE, GRAPE
}
public class FruitMapExample {
public static void main(String[] args) {
EnumMap<Fruit, Integer> fruitQuantities = new EnumMap<>(Fruit.class);
fruitQuantities.put(Fruit.APPLE, 10);
fruitQuantities.put(Fruit.BANANA, 20);
// Accessing a value
System.out.println("Apples: " + fruitQuantities.get(Fruit.APPLE));
// Removing a value
fruitQuantities.remove(Fruit.BANANA);
// Iterating over entries
for (Fruit fruit : fruitQuantities.keySet()) {
System.out.println(fruit + ": " + fruitQuantities.get(fruit));
}
}
}
In this snippet, we demonstrate how to access, remove, and iterate over entries in an EnumMap.
3. Best Practices and Considerations
While EnumMap is powerful, there are some considerations to keep in mind when using it.
3.1 When to Use EnumMap
- Enum Keys Only: Use EnumMap exclusively when your map's keys are enums. It is not suitable for other types of keys.
- Fixed Set of Keys: EnumMap is ideal when the set of keys is fixed and known at compile time.
3.2 Limitations
- Not for Dynamic Keys: If you need a map where keys are not known at compile time or can change, EnumMap is not appropriate.
- No Null Keys: EnumMap does not allow null keys, so you need to handle null values carefully.
4. Conclusion
EnumMap is a robust and efficient map implementation in Java tailored for use with enums. Its performance benefits and type safety make it a valuable tool for managing mappings with a fixed set of keys. By understanding its features and best practices, you can leverage EnumMap to write more efficient and readable code.
Feel free to leave any questions or comments below!
Read more at : Understanding EnumMap in Java: Benefits, Usage, and Examples